- Python Pdb Cheat Sheet
- Python Pdb Commands
- Python Pdb Cheat Sheet
- Python String Manipulation Cheat Sheet
- Cheat Sheets For Python
Python debugger gui
Ipdb is an enhanced version of pdb (built-in). It supports all pdb commands and is simply easier to use like tab for auto-complete. Install: pip install ipdb. Ipdb command cheatsheat. Check here for more commands.
- The module pdb defines an interactive source code debugger for Python programs. It supports setting (conditional) breakpoints and single stepping at the source line level, inspection of stack frames, source code listing, and evaluation of arbitrary Python code in the context of any stack frame.
- Python has a PDB and PHP counterpart is XDebug. Both the debugging tools provide you with standard features like stacks, breakpoints, path mapping, and so on. For this reason, it is hard to decide on which language is better in terms of debuggers.
- Pymol foobar.pdb foobar.pml. But now let get into it and collect some commands. To load in a new pdb code simply use. Fetch pdb-code Selection & Extracting. Pymolwiki on Selection. Sourceforge Pymol Manual. Selection allows you uniquely label a set of atoms that are part of an object (e.g. A protein that you loaded in).
Python debugger, and has some powerful features like object browser, windows for variables, classes, functions, exceptions, stack, conditional breakpoints, etc. Debugger for Python programs with a graphical user interface. It uses bdb (part of stdlib) but adds a GUI and has some powerful features like object browser, windows for variables, classes, functions, exceptions, stack, conditional breakpoints, etc.
pudb · PyPI, A full-screen, console-based Python debugger. Its goal is to provide all the niceties of modern GUI-based debuggers in a more lightweight and The module pdb defines an interactive source code debugger for Python programs. It supports setting (conditional) breakpoints and single stepping at the source line level, inspection of stack frames, source code listing, and evaluation of arbitrary Python code in the context of any stack frame.
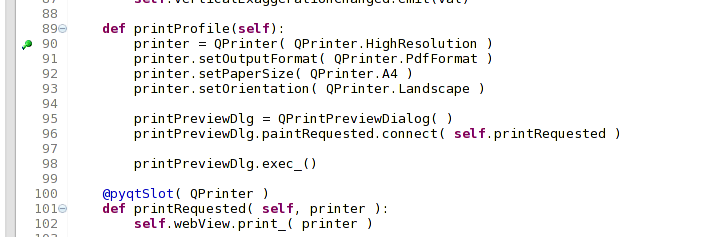
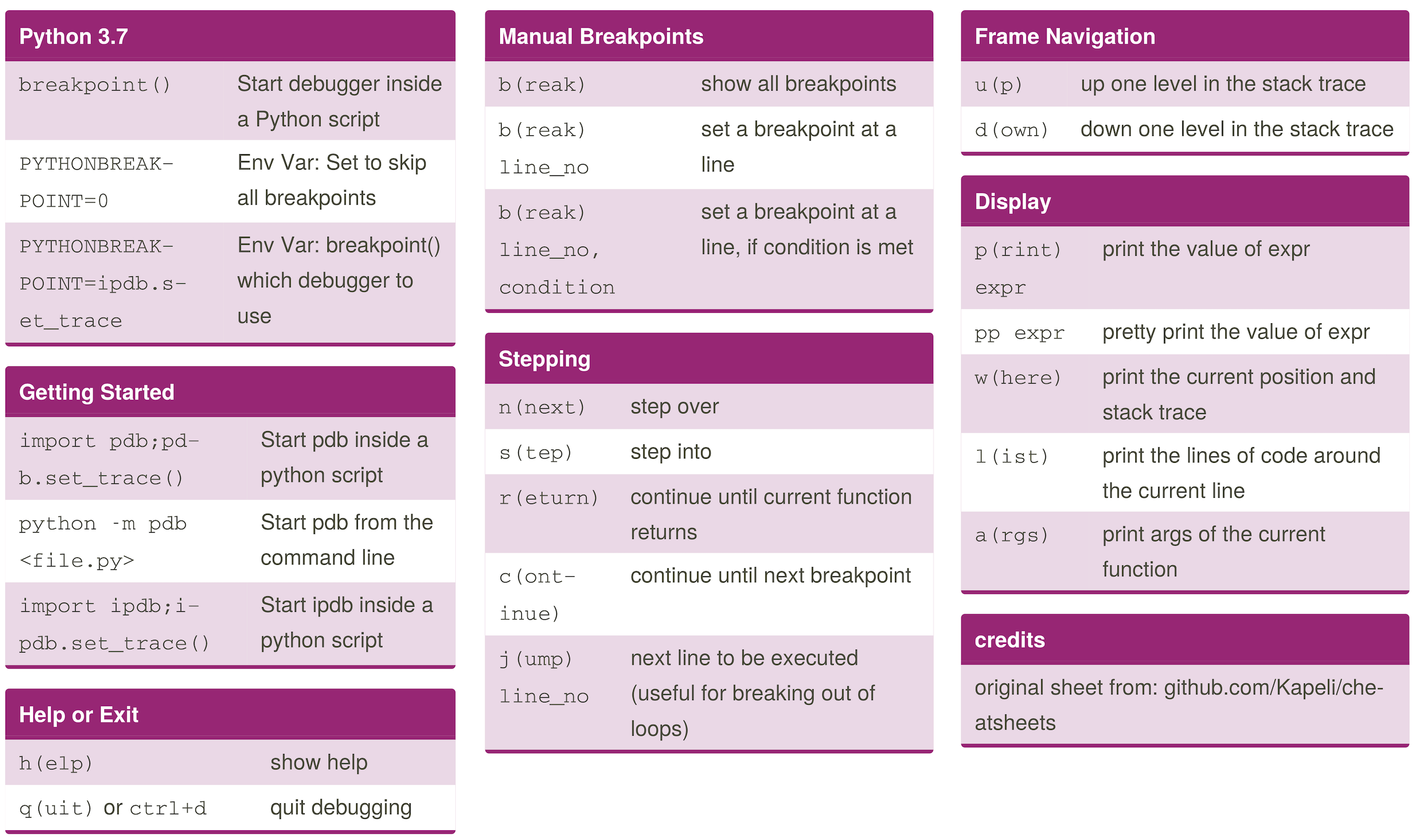

Python Debugger Showdown, Which Python debugger is the best for you? Modern debuggers are GUI-based, and typically either part of an Integrated Development pdb is part of Python’s standard library, so it’s always there and available for use. This can be a life saver if you need to debug code in an environment where you don’t have access to the GUI debugger you’re familiar with. The example code in this tutorial uses Python 3.6. You can find the source code for these examples on GitHub.
Python debugger online
Online Python Debugger - online editor, OnlineGDB is online IDE with python debugger. Easy way to debug python program online. Debug with online pdb console. Online Python Debugger. Code, Run and Debug Python program online. Write your code in this editor and press 'Debug' button to debug program.
Python Debugger, Python Debugger · Image Manipulation · Od Sandbox · Github Repo. Logout. ⏏ Start Debuger ⇤ Back ⇥ Step □ Stop ▷ Run. Code. Console. Write and run Python code using our online compiler (interpreter). You can use Python Shell like IDLE, and take inputs from the user in our Python compiler.
Python Pdb Cheat Sheet
Visualize Python, Java, JavaScript, C, C++, Ruby , Create test cases. These examples demonstrate the tool's visualization capabilities but are not meant as coding lessons. Python Examples. Basic: hello | happy Python statements can also be prefixed with an exclamation point (!). This is a powerful way to inspect the program being debugged; it is even possible to change a variable or call a function. When an exception occurs in such a statement, the exception name is printed but the debugger’s state is not changed. The debugger supports aliases. Aliases can have parameters which allows one a certain level of adaptability to the context under examination.
Python debug flag
Debug Your Python Application, Get Instant Insights Into Python App Performance With End-To-End, Distributed Tracing. Show activity on this post. I would like to have a local debug flag defined in every module of my project and to use it in combination with debug variable in order to be able to do partial debugging. For example f i want to debug module mod1 i would define. mod1_dflag = True.
Python Pdb Commands
Python equivalent for #ifdef DEBUG, The main advantage of a preprocessor compared to setting a DEBUG flag and running code if (DEBUG True) is that conditional checks also Python's re.DEBUG Flag Eric Holscher points out a Python gem I never knew about. If you pass in the number 128 (or, as I have a preference for flags in hex, 0x80) as the second arg to re.compile, it prints out the parse tree of the regex:
1. Command line and environment, Note that, unlike the corresponding Python 3.x flag, this will not emit warnings Turn on parser debugging output (for wizards only, depending on compilation re.DEBUG: If you use this flag, Python will print some useful information to the shell that helps you debugging your regex. re.IGNORECASE: If you use this flag, the regex engine will perform case-insensitive matching. So if you’re searching for [A-Z], it will also match [a-z]. re.I: Same as re.IGNORECASE : re.LOCALE: Don’t use this flag — ever.
How to debug python code
Python debug configurations in Visual Studio Code Initialize configurations #. A configuration drives VS Code's behavior during a debugging session. Configurations are Additional configurations #. By default, VS Code shows only the most common configurations provided by the Python Basic
now - timestamp[0])) timestamp[0] = now #2. debug('Entering nasty piece of code') sleep(.3) debug('First step done.') sleep(.5) debug('Second step done.') This is a bit trickier, but still quite simple. First, you import the time function from the time module in the debug function.
Python Pdb Cheat Sheet
Part 1. Debugging Python Code Preparing an example. Do you remember the quadratic formula from math class?This formula is also known as the A, B, C formula, it’s used for solving a simple quadratic equation: ax2 + bx + c = 0.
Debugging in python tutorial point
The Python Debugger (pdb), Python's standard library contains pdb module which is a set of utilities for debugging of Python programs. The debugging functionality is defined in a Pdb class. The module internally makes used of bdb and cmd modules. To see list of all debugger commands type 'help' in front of the debugger prompt. Start debugging this module from command line. In this case the execution halts at first line in the code by showing arrow (->) to its left, and producing debugger prompt (Pdb) C:python36>python -m pdb fact.py > c:python36fact.py(1)<module>() -> def fact(x): (Pdb) To see list of all debugger commands type 'help' in front of the debugger prompt. To know more about any command use 'help <command>' syntax.
Debugging Thread Applications, In computer programming, debugging is the process of finding and removing the bugs, The Python debugger or the pdb is part of the Python standard library. The script will execute until a certain point; until where a line has been set. Debugging is harder for complex systems in particular when various subsystems are tightly coupled as changes in one system or interface may cause bugs to emerge in another. Debugging is a developer activity and effective debugging is very important before testing begins to increase the quality of the system.
Learn how to debug in Python [Tutorial], Learn how to debug in Python [Tutorial] or inserting print statements at certain points to print the value of variables or points while debugging. If you’re using Python 3.7 or later, I encourage you to use breakpoint () instead of pdb.set_trace (). You can also break into the debugger, without modifying the source and using pdb.set_trace () or breakpoint (), by running Python directly from the command-line and passing the option -m pdb.
Python debugger vscode
Debugging configurations for Python apps in Visual Studio Code, The March 2020 update to Microsoft's wildly popular Python extension for Visual Studio Code focused on improving quality via bug fixes, but it By default, the debugger uses the same python.pythonPath workspace setting as for other features of VS Code. To use a different interpreter for debugging specifically, set the value for python in launch.json for the applicable debugger configuration. Alternately, select the named interpreter on the Status Bar to select a different one.
Get Started Tutorial for Python in Visual Studio Code, Although Visual Studio Code is capable of debugging most of the programming languages, we will use Python in this tutorial. Debug Python This configuration contains 'module': 'flask', which tells VS Code to run Python with -m flask when it starts the debugger. It also defines the FLASK_APP environment variable in the env property to identify the startup file, which is app.py by default, but allows you to easily specify a different file.
Python in Visual Studio Code, Debugging a standard python application is possible by adding the standard to print all output from the program into the VSCode debugger output window. I'm using VSCode in order to debug a Python script. Following this guide I setup the argument in the launch.json file . But when I press on Debug it says that my argument is not recognized: As VSCode is using PowerShell, let's execute the same file with the same argument: So: same file, same path, same argument. In the terminal is working, in
Python pdb cheat sheet
Python Debugger Cheatsheet Getting started startpdbfromwithinascript: importpdb;pdb.set_trace() startpdbfromthecommandline: python-mpdb<file.py> Basics h(elp) printavailablecommands
pdb cheatsheet Invoking the debugger Enter at the start of a program, from the command line: python –m pdb mycode.py Enter in a statement or function: import pdb # your code here if __name__ '__main__': # start debugger at the beginning of a function pdb.runcall(function[, argument, ]) # execute an expression (string) under the debugger
Python Debugger Enjoy this cheat sheet at its fullest within Dash, Start pdb inside a python script. python -m pdb <file.py> Start pdb from the command line.
Stepping through a python script
Python String Manipulation Cheat Sheet
How to step through Python code to help debug issues?, You can then step through the code following this statement, and continue running without the debugger using the c command. The typical usage The buttons you’ll see in this lesson allow you to debug and run a script step by step in order to see what’s happening. These step buttons are really powerful because they allow you to see what’s going on under the surface.
26.2. pdb — The Python Debugger, You can then step through the code following this statement, and continue running without the debugger using the continue command. New in version 3.7: The Technically, the PVM is the last step of what is called the Python interpreter. The whole process to run Python scripts is known as the Python Execution Model. Note: This description of the Python Execution Model corresponds to the core implementation of the language, that is, CPython.
pdb — The Python Debugger, There are two commands you can use to step through code when debugging: n stands for next. It allows you to move to the next logically executed line of code, A PythonScriptStep is a basic, built-in step to run a Python Script on a compute target. It takes a script name and other optional parameters like arguments for the script, compute target, inputs and outputs. If no compute target is specified, the default compute target for the workspace is used.
Cheat Sheets For Python
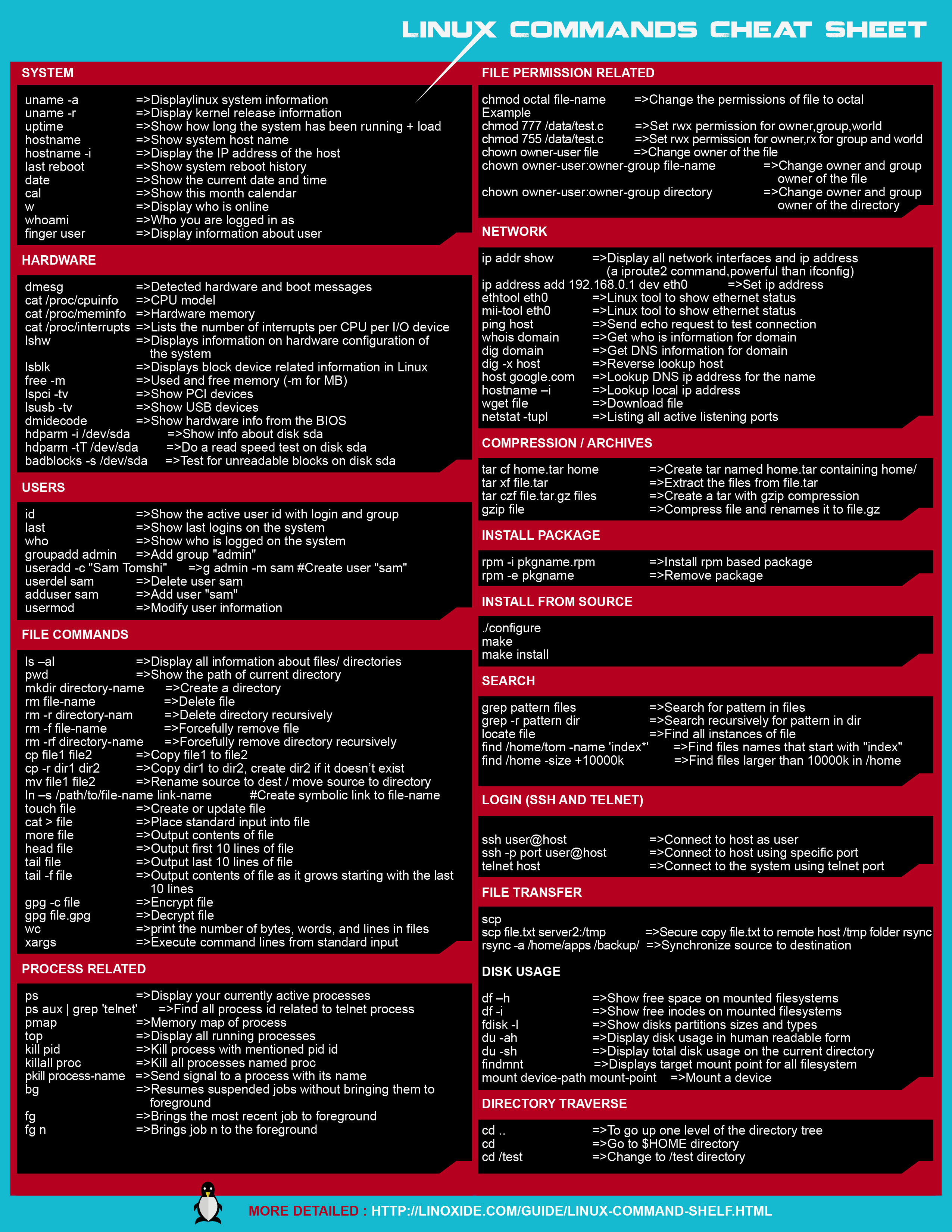
More Articles
